This is first of 4 post series to introduce you to OO ABAP which is enough to start adopting OO ABAP in case you have not done so so far.
In this series, you will learn below –
- Introduction to ABAP Class
- More on ABAP Class
- Triggering And Handling Events
- Exception Handling
We will be using Eclipse IDE to create and work with classes. It is easier in Eclipse as both local and global classes are created in source code editor.
Related Video:
Create a class in Eclipse
Right click on the the project / package and select New->ABAP Class.
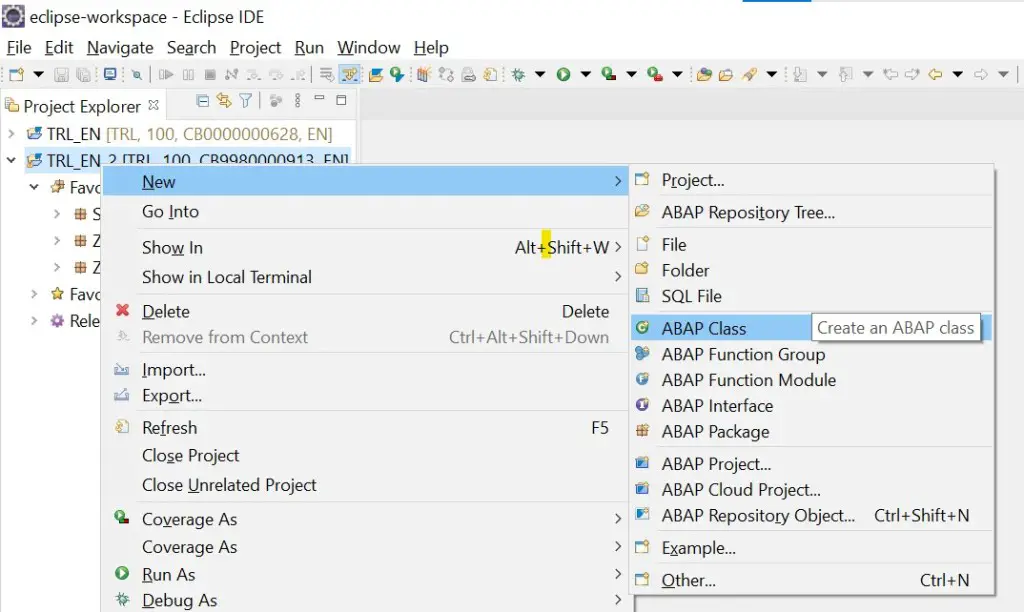
Provide class name and Description for the class and click next. Provide a TR and click Finish.
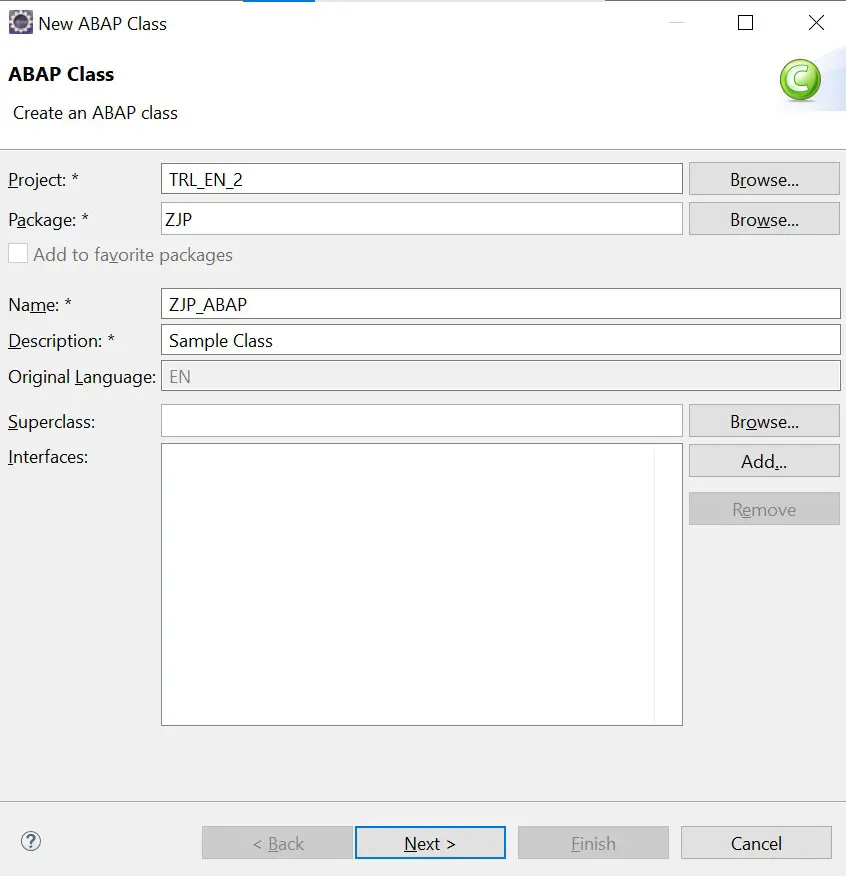
Below definition is created.
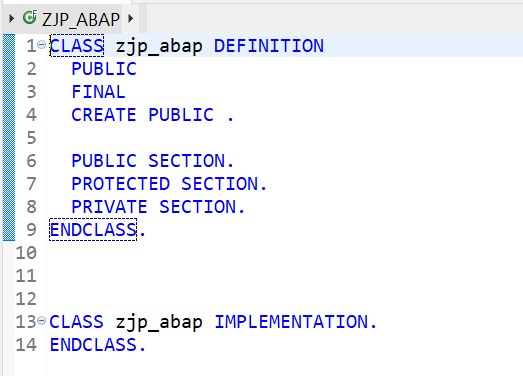
Class Structure
Below is a typical class structure –
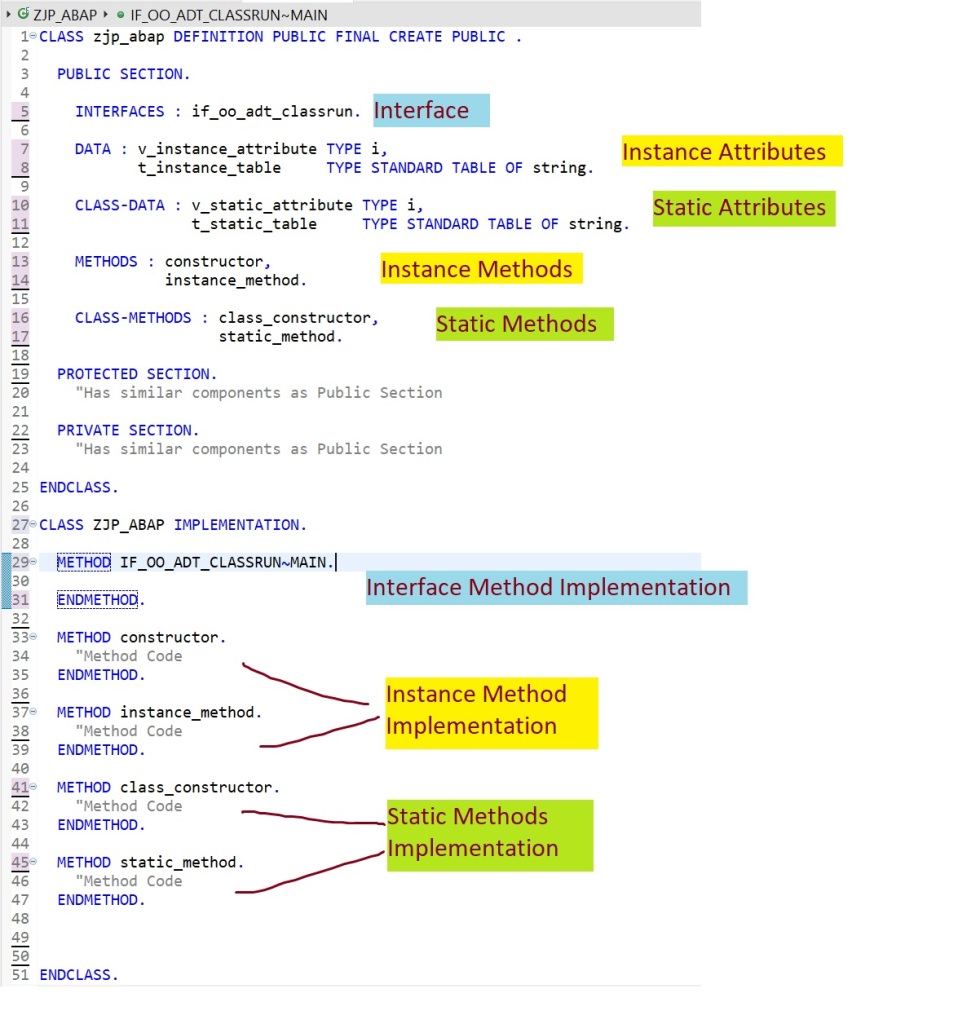
Class Definition
- A class is defined with key word CLASS followed by class name and key word DEFINITION.
- Using PUBLIC after DEFINITION makes the class global.
- FINAL means the class can not be extended by way of inheritance.
- CREATE PUBLIC means the class can be instantiated from anywhere (from any other class or program or subroutine etc)
A class has 3 visibility sections – PUBLIC, PROTECTED and PRIVATE.
- PUBLIC – The attributes and methods defined in this section can be accessed inside and outside the class.
- PROTECTED – The attributes and methods defined in this section can be accessed inside the class and its subclasses
- PRIVATE – The attributes and methods defined in this section can be accessed only inside the class itself.
Attributes – The data within the class. It can be variable, structure or tables.
DATA :
v_instance_attribute TYPE i,
t_instance_table TYPE STANDARD TABLE OF string.
CLASS-DATA :
v_static_attribute TYPE i,
t_static_table TYPE STANDARD TABLE OF string.
Methods – These are block of codes within the class which can be called to perform certain function.
METHODS :
constructor,
instance_method.
CLASS-METHODS :
class_constructor,
static_method.
Instance Attributes/Methods
- Instance attributes are defined with DATA
- These are specific to each instance of a class
- Instance methods are defined with METHODS
- Instance methods can access instance as well as static attributes/methods
Static Attributes/Methods- Instance attributes are defined with DATA, instance methods are defined with METHODS
- Static attributes are defined with CLASS-DATA
- These are not specific to each instance of a class and are common across all instances of a class
- Static methods are defined with CLASS-METHODS
- Static methods can only access static attributes
Constructor
Special instance method that is called automatically when instance of a class is created.
METHODS : constructor.
Class Constructor
Special static method that is called automatically when any static attribute/method is accessed for the first time.
CLASS-METHODS : class_constructor.
Interface
Interfaces have attributes and method definitions. Method implementations are not part of the interfaces and have to be implemented within the class implementation section. These are reusable components.
INTERFACES : if_oo_adt_classrun.
Example of an interface.
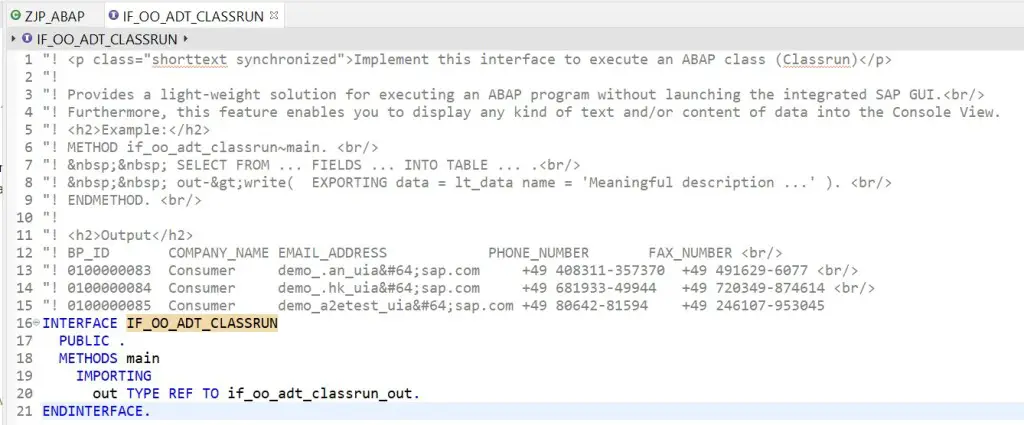
Class Implementation
Let us look at the class definition one more time. This time only the elements which require implementation. Attributes are removed from below code for simplicity.
CLASS zjp_abap DEFINITION PUBLIC FINAL CREATE PUBLIC .
PUBLIC SECTION.
INTERFACES : if_oo_adt_classrun.
METHODS : constructor,
instance_method.
CLASS-METHODS : class_constructor,
static_method.
ENDCLASS.
So, what we need to implement is the methods within the interface added and the methods in the class. Without actual code inside the methods, the implementation part will look like below.
CLASS zjp_abap IMPLEMENTATION.
METHOD if_oo_adt_classrun~main.
"Method Code
ENDMETHOD.
METHOD constructor.
"Method Code
ENDMETHOD.
METHOD instance_method.
"Method Code
ENDMETHOD.
METHOD static_method.
"Method Code
ENDMETHOD.
METHOD class_constructor.
"Method Code
ENDMETHOD.
ENDCLASS.
For more posts on Object Oriented ABAP, please visit page OOABAP.