In this post, you will learn about Concurrency handling in ABAP RESTful Application Programming.
Concurrency
- Concurrency handling means handling simultaneous update requests for the same resource
- If simultaneous update requests are received, the service should allow only one update at a time and reject other updates
Concurrency needs to be managed as we need to prevent concurrent and interfering database access of different users and ensures that data can only be changed if data consistency is assured.
Ways to manage concurrency
- Pessimistic Concurrency Control (Locking)– assume that concurrent writes will occur and hence protects it by aggressively locking out resources.
- Optimistic Concurrency Control– assumes that the likelihood of a concurrent write is rare and so allows the operation to continue and only check at the final moment while updating the data
Optimistic Concurrency Control
This approach uses a etag field. The etag field usually contains a timestamp, a hash value, or any other versioning that precisely identifies the version of the data set.
- READ operation sends etag field
- UPDATE operation checks whether the etag matches, if the etag does not match it means that the entity is changed already by someone else and the UPDATE operation is rejected.
They way this works is similar to service built using SEGW and below posts cover those scenarios.
In RAP scenario, the etag field is mentioned in the Behavior Definition.
Usually, the Parent or the root entity is the etag master and the child is etag dependent entity.
etag master MasterField
- Defines entity as etag master
- Managed RAP BO – a value for the total etag field can be provided automatically if the etag field is annotated in the CDS with
@Semantics.systemDateTime.localInstanceLastChangedAt: true
and the data type is compatible with date. - Unmanaged RAP BO – developer must provide/update the etag field in all MODIFY_ENTITY operations.
If the etag is not automatically updated, then it should be treated similar to unmanaged scenario.
etag dependent by _Assoc
- Defines an entity as etag dependent. This entity does not have its own etag field and uses the etag field of the etag master entity

total etag TotalEtagField
When we have a draft enabled scenario, we need to specify total etag field as well.
- Defines a field as total ETag field for draft-enabled scenarios
- This enables optimistic concurrency checks during the transition from draft to persistent data
- The total etag field has to be defined in the lock entity (defined by lock master)
- The field that is specified as TotalEtagField must be annotated in CDS with the annotation
@Semantics.systemDateTime.lastChangedAt: true
and the data type must be date compatible.

The annotation mentioned in the above explanation look like below in CDS view. Note that localInstanceLastChangedAt
is for etag master and the total etag and the annotation lastChangedAt
is for the total etag.
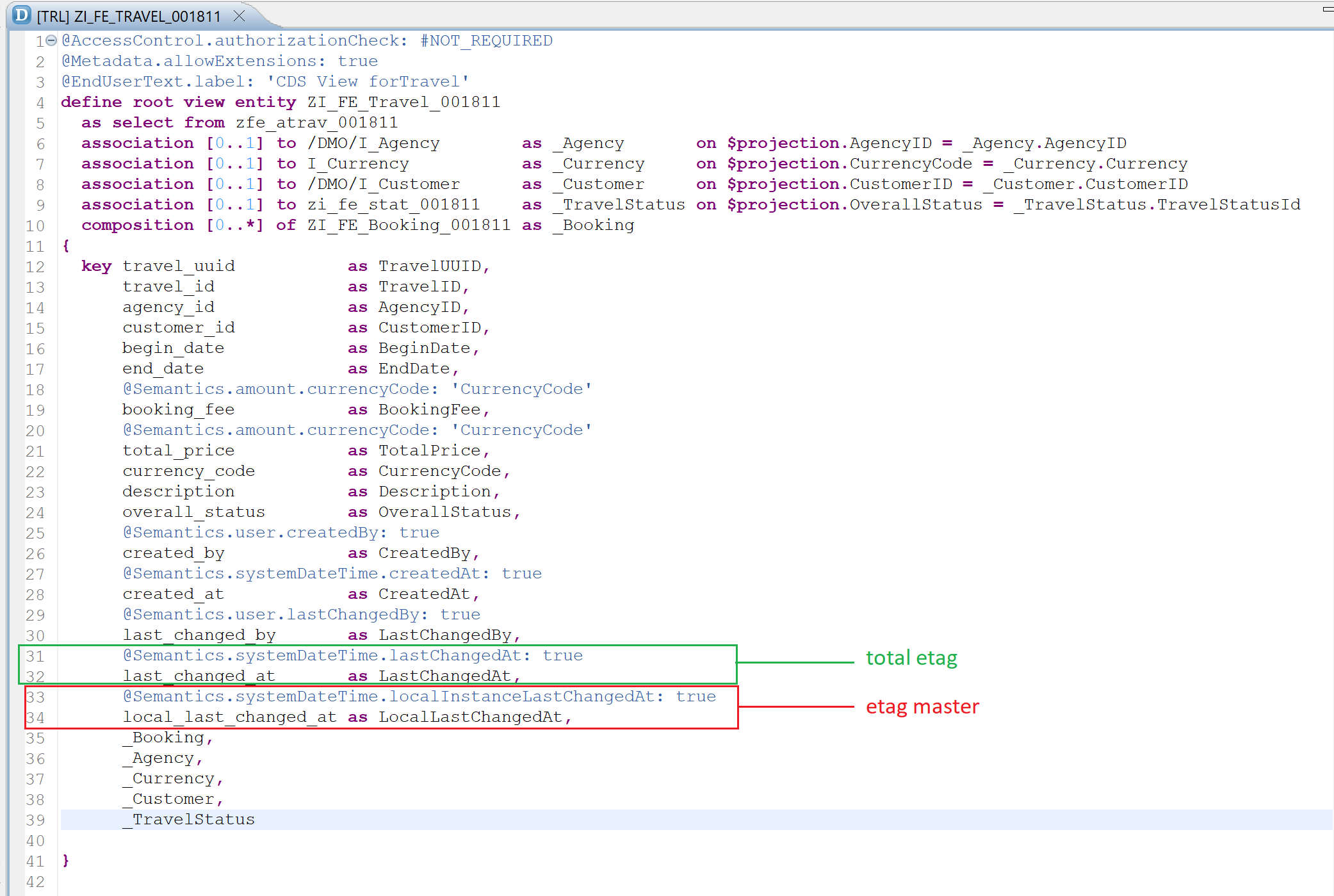
How total etag works in RAP BO?
- This field is updated by RAP runtime framework as soon as an active instance is changed. This refers to the entire BO and not just one entity.
- The total etag value is compared when resuming a draft instance to ensure that the active data has not been changed after the exclusive lock has expired.
Pessimistic Concurrency Control (Locking)
Pessimistic concurrency control uses exclusive lock on the data. So, the data being modified by one user can not be modified by other users at the same time.
The lifetime of such an exclusive lock is affected by multiple factors –
- ABAP session: The enqueue lock is released once the ABAP session is terminated.
- LUW: The lock is released, once the RAP LUW is terminated with a COMMIT or ROLLBACK.
- Draft: In draft scenarios, the lock is not coupled to the LUW or the ABAP session, but depends on the lifetime of the draft instance. The lock is released if the draft is discarded or draft is activated i.e. saved as active instance.
Locking in Non-Draft Scenarios
Lock is invoked in operations
- update,
- delete,
- create by association, and
- action
Create operation does not invoke lock as there is no active instance to lock.
Managed Scenarios
The RAP framework assumes all of the locking tasks.
Unmanaged Scenarios
The application developer has to implement the method for lock and implement the locking mechanism including the creation of the lock object.
Locking in Draft Scenarios
- As soon as a draft instance is created for an existing active instance, the active instance receives an exclusive lock and cannot be modified by another user.
- This exclusive lock remains for a determined time, even if the ABAP session terminates.
- The duration time of the exclusive lock can be configured. Once the exclusive lock expires after this duration time, the optimistic lock phase begins. The phase ends when,
- the lock owner (user) discards the draft explicitly
- draft is discarded implicitly (draft is discarded automatically by the life-cycle service, 28 days by default as per the SAP documentation; or the corresponding active instance is changed directly without using the draft; or other user requests exclusive lock
- The draft is resumed by the draft owner – here the optimistic lock phase ends and exclusive lock phase starts again
Defining Lock in RAP BO
The lock is defined in the behavior definition.
lock master [unmanaged] | lock dependent by _AssocToLockMaster
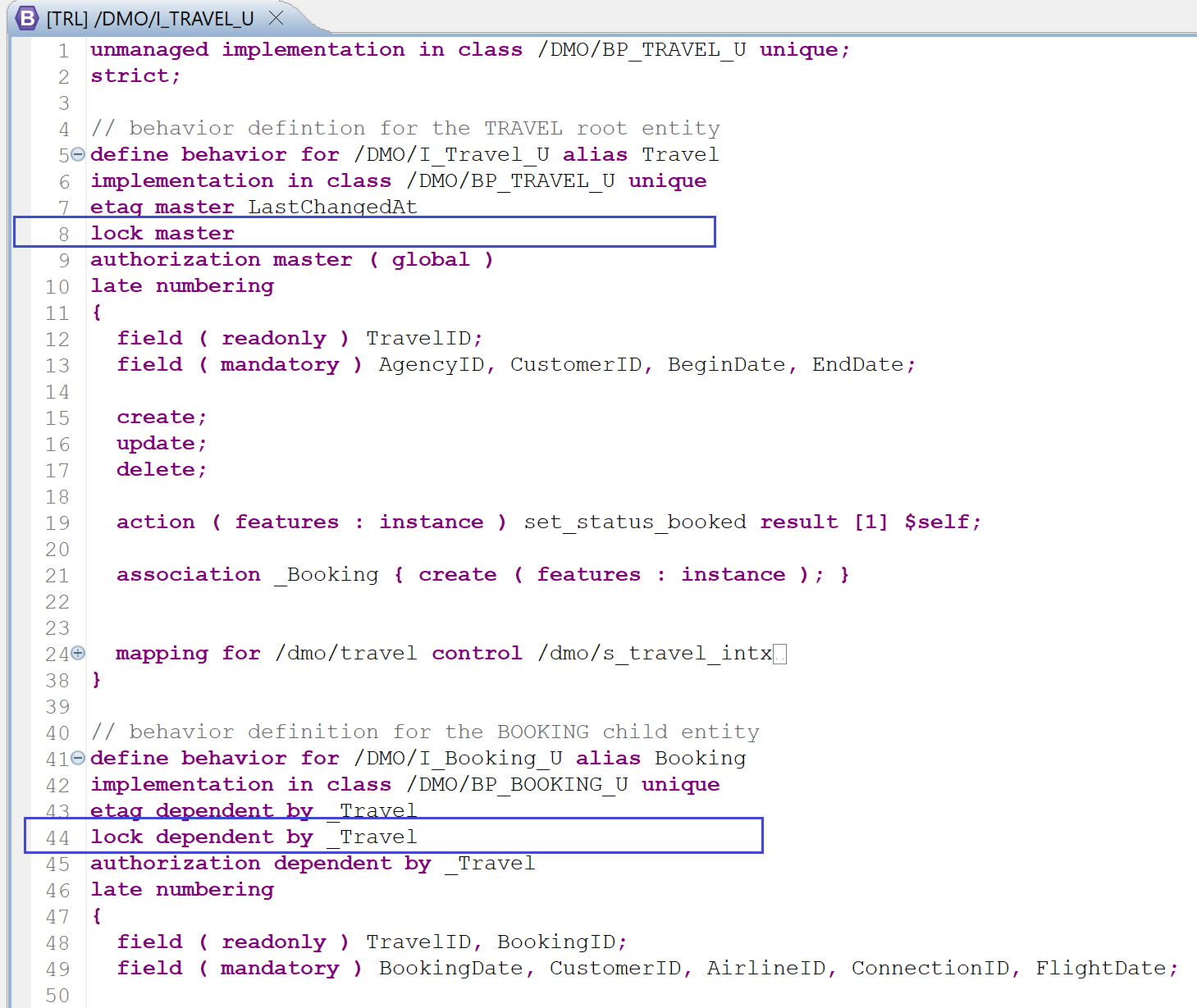
The lock statements variants are as below.
Lock Master
In Managed scenario, the RAP framework handles the locks. Lock master entities are locked on lock request of the master or any lock dependent entity.
In unmanaged scenario, the FOR LOCK method must be implemented. The lock implementation must include the dependent entities as well.
Lock Master Unmanaged
In the managed scenario, lock master can be unmanaged. Here, the FOR LOCK method must be implemented. The lock implementation must include the dependent entities as well.
Lock Dependent
The lock master entity of lock dependent entities is identified via the association to the lock master entity. The lock implementation is delegated to the master.
Sample Implementation of the Lock in Unmanaged Scenario
This can be either unmanaged scenario or managed scenario with unmanaged locks. In both cases the method FOR LOCK needs to be implemented in the behavior pool.
Definition example
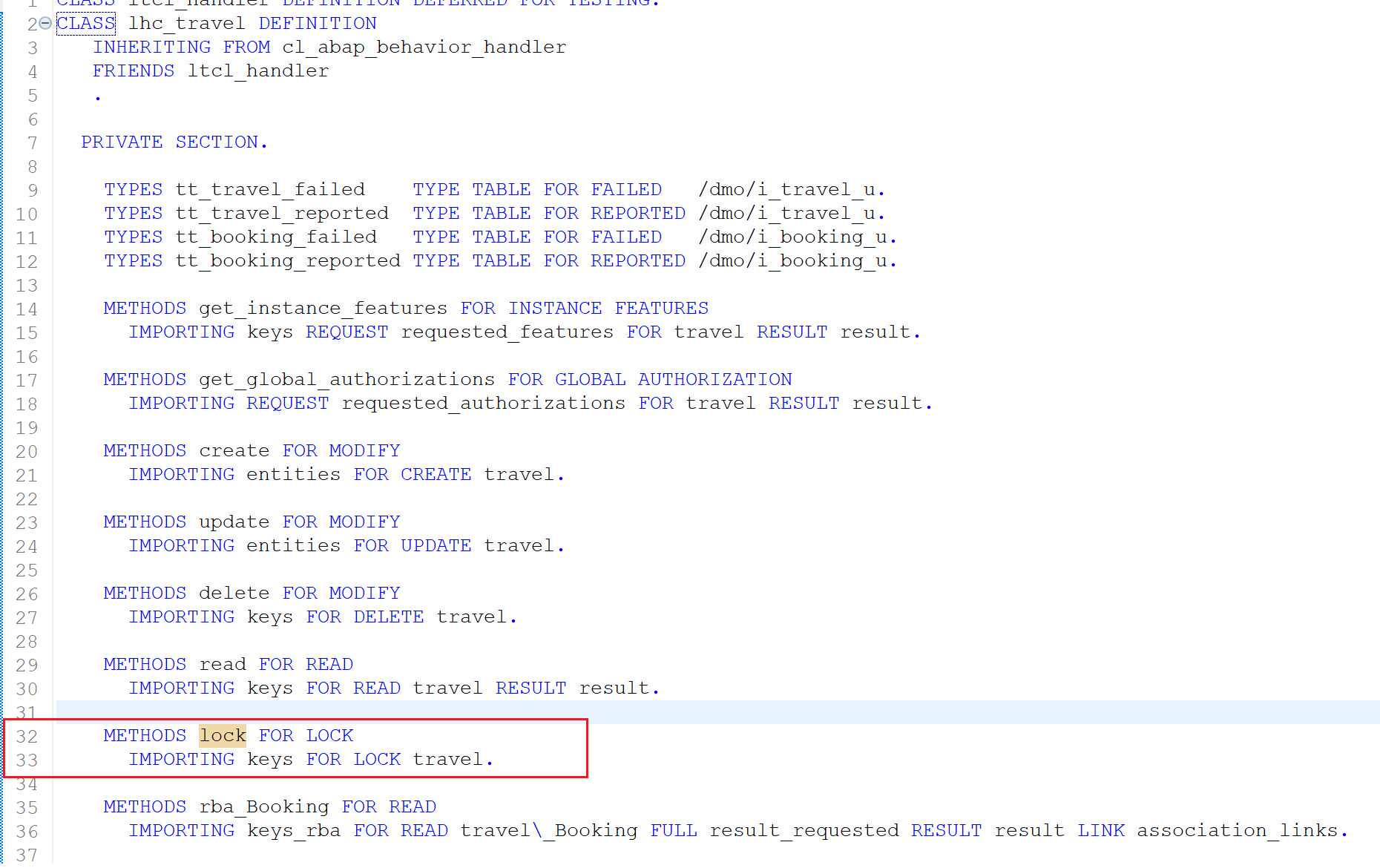
METHODS lock FOR LOCK
IMPORTING keys FOR LOCK travel.
Implementation Example
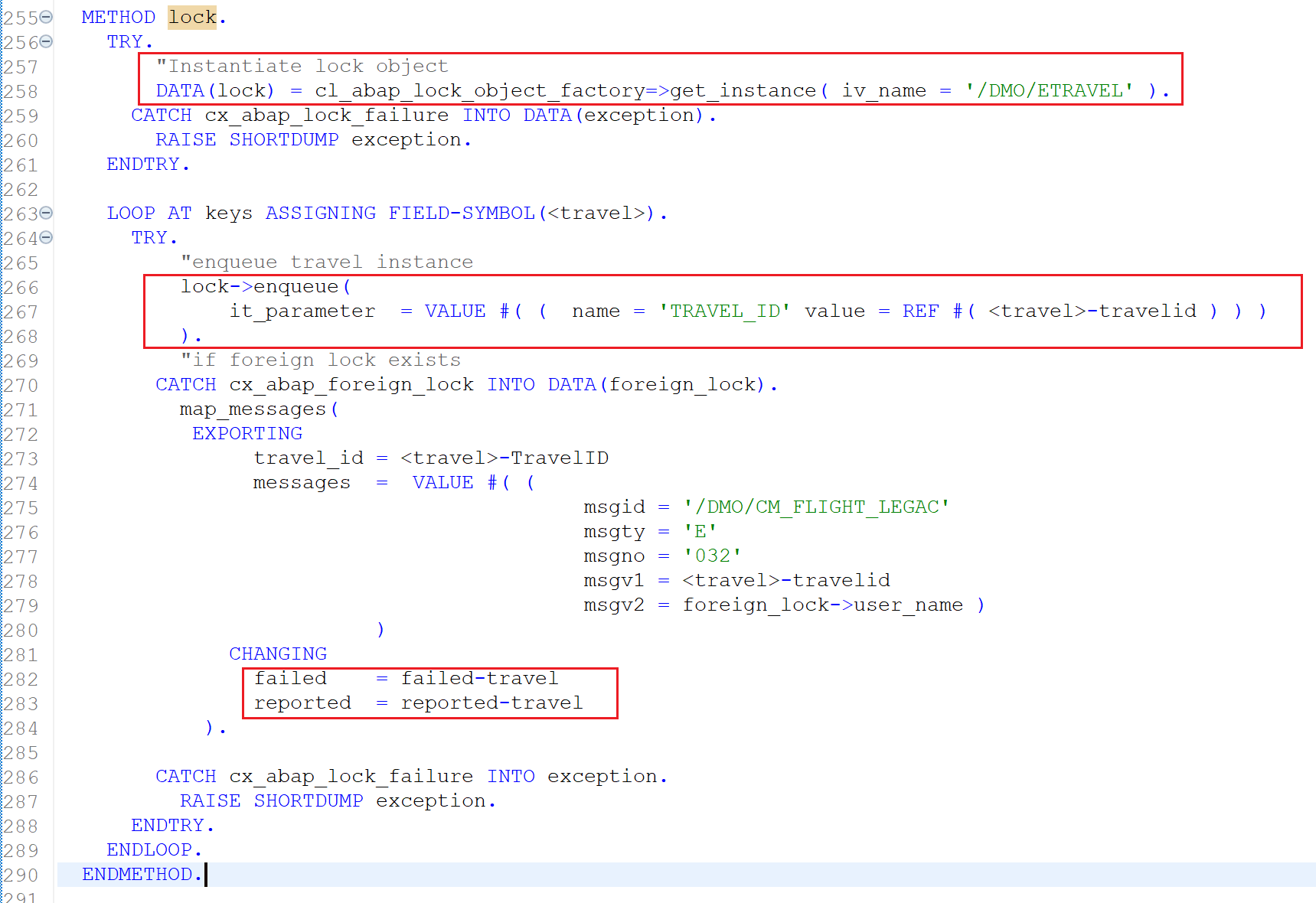
Here, the relevant lock object is /DMO/ETRAVEL.
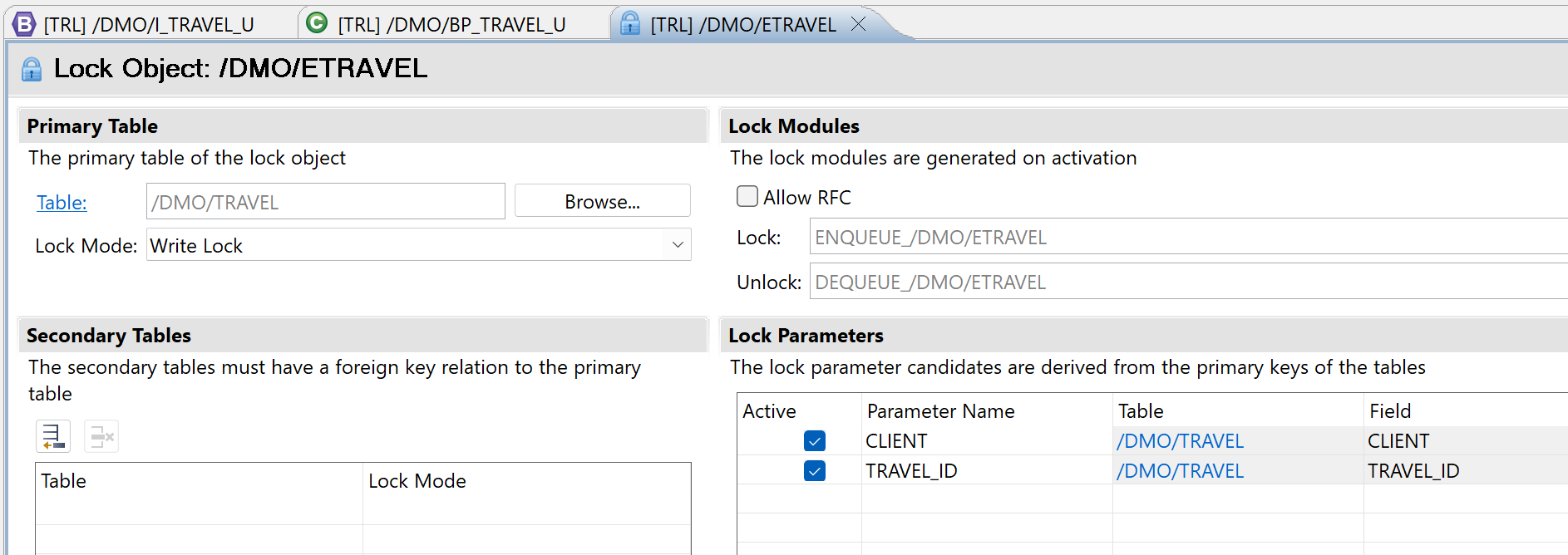
Instead of calling enqueue and dequeue function modules, methods of factory class cl_abap_lock_object_factory
are used.
Sample code for reference
METHOD lock.
TRY.
"Instantiate lock object
DATA(lock) = cl_abap_lock_object_factory=>get_instance( iv_name = '/DMO/ETRAVEL' ).
CATCH cx_abap_lock_failure INTO DATA(exception).
RAISE SHORTDUMP exception.
ENDTRY.
LOOP AT keys ASSIGNING FIELD-SYMBOL(<travel>).
TRY.
"enqueue travel instance
lock->enqueue(
it_parameter = VALUE #( ( name = 'TRAVEL_ID' value = REF #( <travel>-travelid ) ) )
).
"if foreign lock exists
CATCH cx_abap_foreign_lock INTO DATA(foreign_lock).
map_messages(
EXPORTING
travel_id = <travel>-TravelID
messages = VALUE #( (
msgid = '/DMO/CM_FLIGHT_LEGAC'
msgty = 'E'
msgno = '032'
msgv1 = <travel>-travelid
msgv2 = foreign_lock->user_name )
)
CHANGING
failed = failed-travel
reported = reported-travel
).
CATCH cx_abap_lock_failure INTO exception.
RAISE SHORTDUMP exception.
ENDTRY.
ENDLOOP.
ENDMETHOD.
Reference: https://help.sap.com/docs/btp/sap-abap-restful-application-programming-model/concurrency-control
Visit ABAP RESTful Application Programming Model to explore all articles on ABAP RAP Model.
If you like the content, please subscribe…