This post explains the class where the behavior definition methods are implemented. This is covered in various posts of the ABAP RAP Series, however the methods are scattered across the posts.
A business object is implemented through a specialized class pool that references its behavior definition.
When the unmanaged implementation type is used, developers must implement all the behavior. This involves coding for all necessary operations, including create, update, and delete, determinations and validations.
Conversely, with the managed implementation type, the framework handles the standard operations, such as create, update, and delete, while application developers only need to create non-standard behavior components like determinations and validation etc.
The behavior is implemented in a special type of class pool called as the behavior pool. The syntax is as below.
CLASS ClassName DEFINITION
PUBLIC ABSTRACT FINAL
FOR BEHAVIOR OF BehaviorDefinition.
ENDCLASS.
CLASS ClassName IMPLEMENTATION.
ENDCLASS.

This global class is defined as ABSTRACT and FINAL. The actual implementation is in the local classes defined within this class. The implementation is split into interaction phase and a save sequence. Both of these are implemented as local handler classes.
- LCL_HANDLER – This is derived from CL_ABAP_BEHAVIOR_HANDLER and handles the interaction phase.
- LCL_SAVER – This is derived from CL_ABAP_BEHAVIOR_SAVER and handles the save sequence.
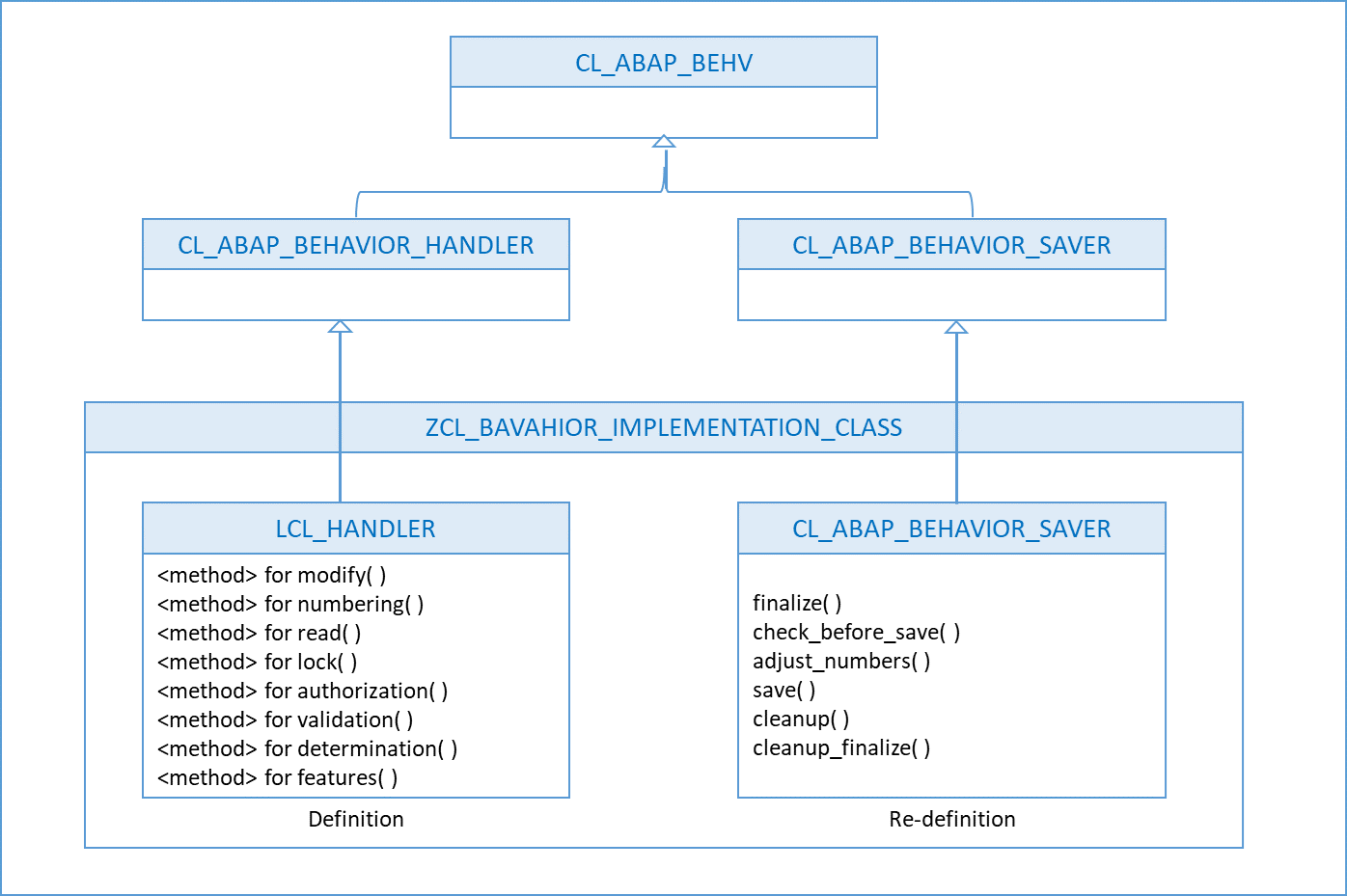
Local Handler Class
The definition of the handler class contains all the methods that are required to implement the behavior.
It is important to note that COMMIT WORK and ROLLBACK WORK are forbidden statements in RAP handler methods.
CLASS lcl_handler DEFINITION INHERITING FROM cl_abap_behavior_handler.
PRIVATE SECTION.
/* FOR MODIFY method declaration */
METHODS modify_method FOR MODIFY
[IMPORTING]
create_import_parameter FOR CREATE entity
update_import_parameter FOR UPDATE entity
delete_import_parameter FOR DELETE entity
action_import_parameter FOR ACTION entity~action_name
[REQUEST requested-fields]
[RESULT action_export_parameter]
create_ba_import_parameter FOR CREATE entity\_association.
/* FOR AUTHORIZATION method declaration */
METHODS auth_method FOR AUTHORIZATION
[IMPORTING] keys REQUEST requested_features FOR entity
RESULT result_parameter.
/* FOR FEATURES method declaration */
METHODS feature_ctrl_method FOR FEATURES
[IMPORTING] keys REQUEST requested_features FOR entity
RESULT result_parameter.
/* FOR GLOBAL FEATURES method declaration */
METHODS get_global_features FOR GLOBAL FEATURES
[IMPORTING] REQUEST requested_features FOR entity
RESULT result_parameter.
/* FOR LOCK method declaration */
METHODS lock_method FOR LOCK
[IMPORTING] lock_import_parameter FOR LOCK entity.
/* FOR READ method declaration */
METHODS read_method FOR READ
[IMPORTING] read_import_parameter FOR READ entity
RESULT read_export_parameter.
/* FOR READ by association method */
METHODS read_by_assoc_method_name FOR READ
[IMPORTING] read_ba_import_parameter FOR READ entity\_association
FULL full_read_import_parameter
RESULT read_result_parameter
LINK read_link_parameter.
ENDCLASS.
Method Summary
1. <method> FOR MODIFY
– Handles all changing operations such as create, update, and delete
All operations can be declared in single method or multiple FOR MODIFY methods can be defined.
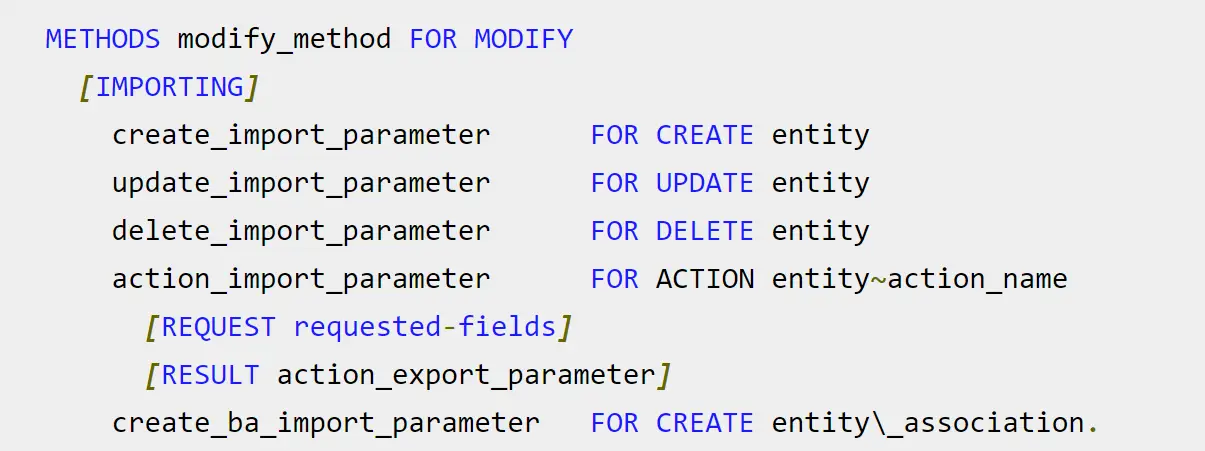
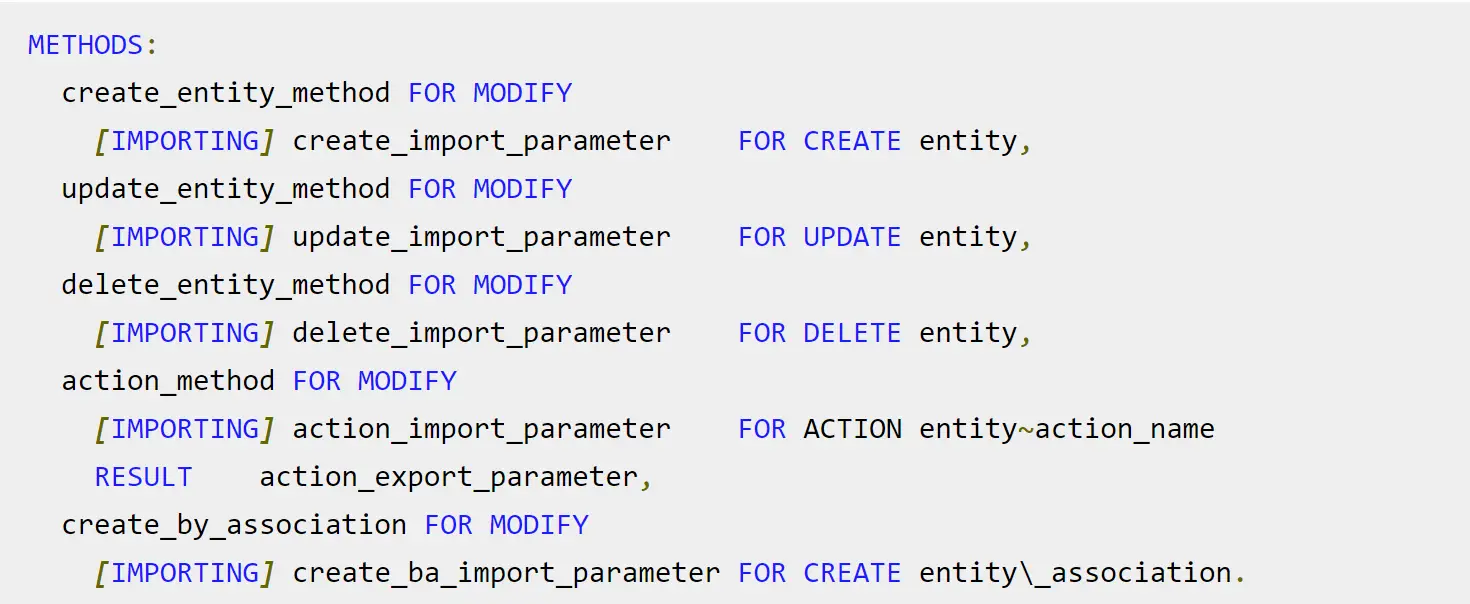
- Importing parameters can not be passed by value
- The method FOR MODIFY has three implicit changing parameters failed, mapped, and reported. These parameters can be explicitly declared for better clarity, but it is not mandatory
2. <method> FOR AUTHORIZATION
– Implements authorization checks

3. <method> FOR FEATURES
– Implements the dynamic feature control of entities

4. <method> FOR GLOBAL FEATURES
– Implements the global feature control

5. <method> FOR LOCK
– Implements the locking of entities

The LOCK method also provides the implicit CHANGING parameters failed and reported.
6. <method> FOR READ
– Handles the processing of reading requests
Declaration of <method> FOR READ

Declaration of <method> FOR READ By Association
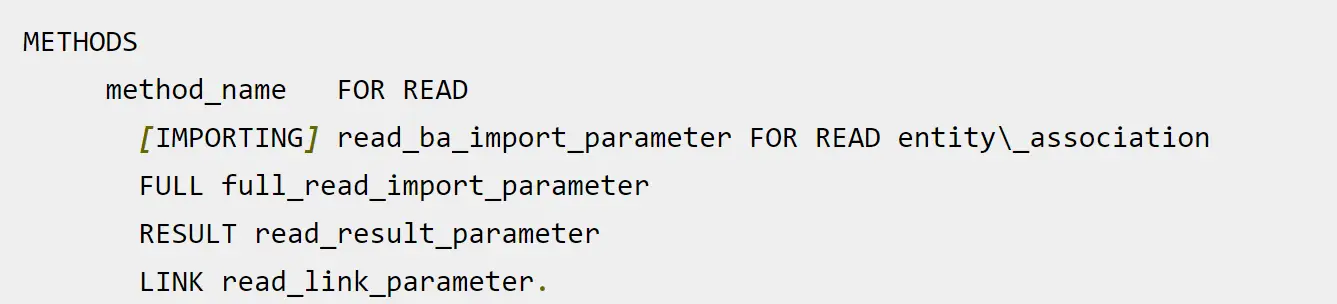
Local Saver Class
The save sequence is called after at least one successful modification was performed using the BO behavior.
Method Details
- FINALIZE – Finalizes data changes before they can be persisted on the database.
- CHECK_BEFORE_SAVE – Checks the transactional buffer for consistency.
- ADJUST_NUMBERS – Implements late numbering.
- SAVE – Saves the data from the transactional buffer to the database.
- CLEANUP – Clears the transactional buffer.
- CLEANUP_FINALIZE – Clears the transactional buffer if the phase FINALIZE or CHECK_BEFORE_SAVE fails.
Derived Data Types
In the behavior implementation the ABAP compiler implicitly derives certain data types from from the involved CDS entities. The derived types contain keys and data for the entities.
The method for an action can be written in two ways,
"Implicit / derived types
METHODS method_name FOR MODIFY IMPORTING keys FOR ACTION action_name.
OR
"Explicit declarations in the definition
METHODS method_name FOR MODIFY IMPORTING keys FOR ACTION action_name
IMPORTING keys TYPE TABLE FOR ACTION IMPORT action_name
CHANGING mapped TYPE RESPONSE FOR MAPPED EARLY root_cds_name
failed TYPE RESPONSE FOR FAILED EARLY root_cds_name
reported TYPE RESPONSE FOR REPORTED EARLY root_cds_name
Derived data types contain fields based on the entity structure and also some additional special fields starting with %
For example, %key summarizes all primary keys. The use of the components depends on the RAP operation and/or context, for example, %pid is only available for late numbering scenarios.
Implicit Response Parameters
These are specific derived types which are derived for Response.
- FAILED – Data set where error has occurred
- REPORTED – Data set where message needs to be displayed
- MAPPED – Used in create scenario to pass back the keys
Visit ABAP RESTful Application Programming Model to explore all articles on ABAP RAP Model.
If you like the content, please subscribe…