One of the important but ignored area in ABAP or OO ABAP is Exception Handling. Most of the developer will just skip implementing error handling using exceptions and rather use exporting or returning parameters in methods to notify that there are errors.
So let us look at how we can change it. Let us start with a way to handle existing exceptions.
TRY-CATCH block
A simple try catch block looks like below.
TRY .
lv_var = 1 / 0.
CATCH cx_sy_zerodivide.
lv_var = 1.
ENDTRY.
A more detailed error handling example using details from exception class is given below.
TRY .
lv_var = 1 / 0.
CATCH cx_sy_zerodivide INTO lo_error.
lv_var = 1.
lv_text = lo_error->if_message~get_text( ).
lo_error->get_source_position(
IMPORTING program_name = lv_prog_name
include_name = lv_incl_name
source_line = lv_source_line ).
"The source code details to be printed/to be added to log
ENDTRY.
So, as you can see, catching exceptions and and getting error details from exceptions are very easy. As all standard SAP exception classes are similar.
Hierarchy of exception classes
Exception classes – Class based exceptions can store more details about the error than simple exceptions that we handle using sy-subrc.
- As all exception classes are derived from CX_ROOT indirectly, they have similar structure and easy to understand.
- You can access any exception object through reference variable of CX_ROOT
- However, new exception class can not be directly inherited from CX_ROOT and its subclasses like CX_NO_CHECK, CX_DYNAMIC_CHECK, CX_STATIC_CHECK should be used to create new classes.
- Important methods: GET_SOURCE_POSITION, GET_TEXT
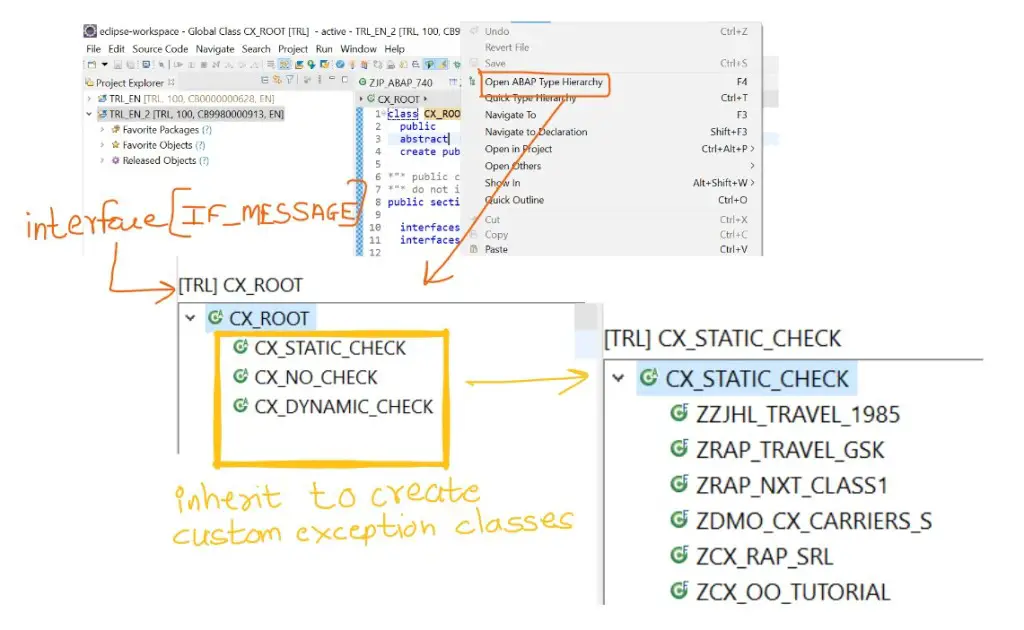
Few important details about these classes are as below.
CX_STATIC_CHECK
- Handle the exception or propagate
- Syntax error if not handled or propagated
- Global exception class is by default inherited from CX_STATIC_CHECK
CX_DYNAMIC_CHECK
- No warning if not handled or propagated
- Runtime error if exception is raised at runtime and is not handled
CX_NO_CHECK
- Can not be propagated explicitly, if not handled, these are auto-propagated till the top level
- Runtime error if not handled by any level
Create new exception class
To create a new exception class, we can inherit any one of CX_NO_CHECK, CX_DYNAMIC_CHECK, CX_STATIC_CHECK i.e. while creating the new class one of these classes have to be specified as the Superclass of the new class.
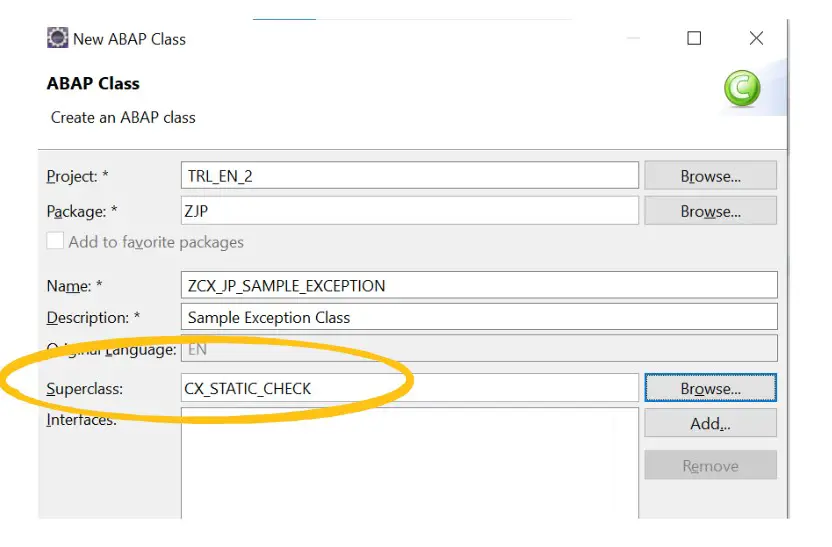
When such class is created, below code will be created automatically. Notice that the methods call superclass methods by default and class specific code can be added here.
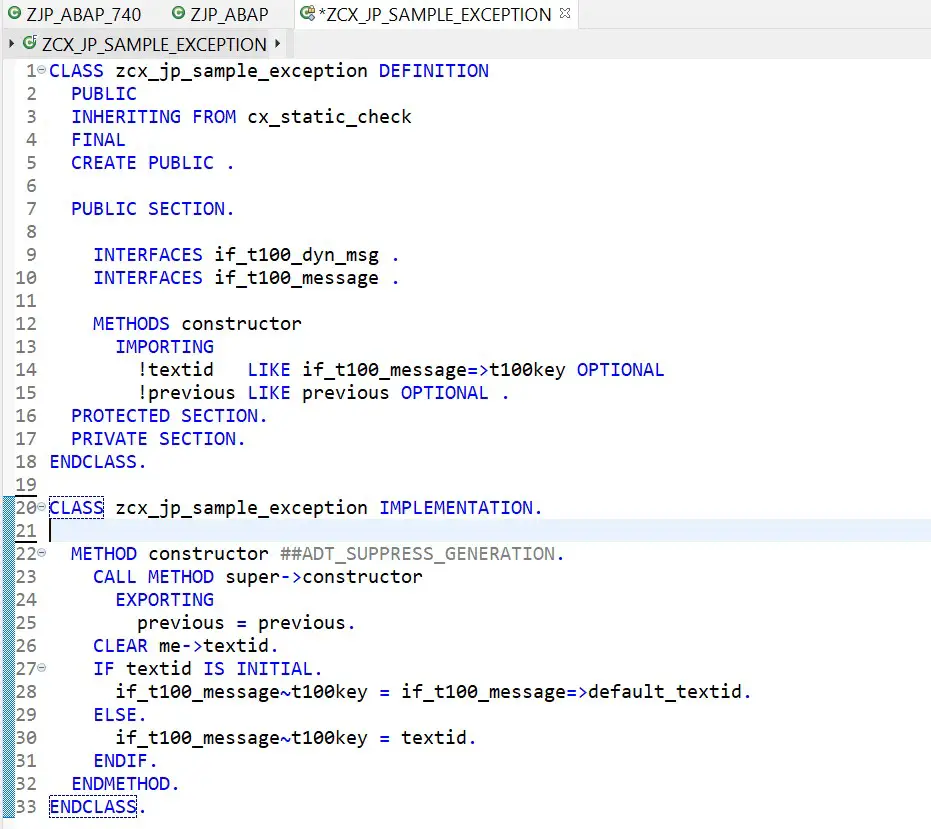
Use the new exception class
To raise exception using a new class, simply use below statement.
RAISE EXCEPTION TYPE zcx_jp_sample_exception.
You have to declare in the method that this exception is going to be triggered, and handle the exception where the method is called. The overall code at various places will look like below.
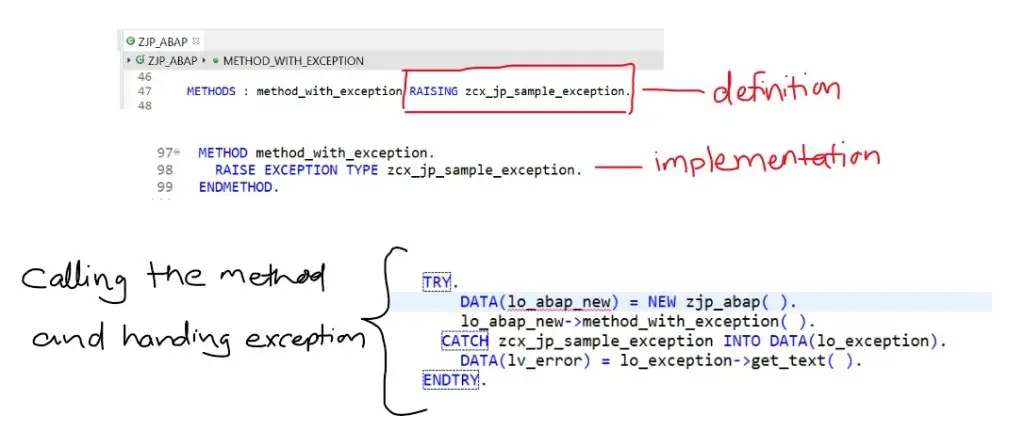
Most of the time, you will not need to create exception class, but only to handle the exceptions using TRY…CATCH block and make use of the exception class methods to get the correct details about the error.
For more posts on Object Oriented ABAP, please visit the page OOABAP.
If you like the content, please subscribe…